Python Algorithm for File Updates
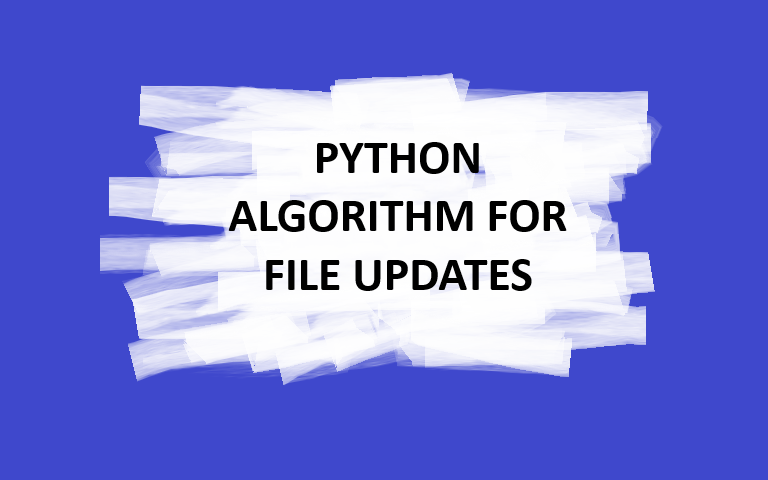
Algorithm for file updates in Python
Project description
An allowed list of IP addresses is used at my organization to manage access to restricted content. The "allow_list.txt" file contains information on these IP addresses. A separate remove list indicates the IP addresses that should no longer have access to this content. I developed an algorithm to automatically update the "allow_list.txt" file and remove IP addresses that should no longer have access.
Open the file that contains the allow list
I opened the "allow_list.txt" file for the first part of the algorithm. I started by setting the import_file variable to contain this file's name:
Subsequently, I used a with statement to access the file:
The algorithm uses the with statement together with the .open() function in read mode to access the allow list file for reading purposes. The file is opened to facilitate access to the IP addresses contained within the allow list file. The with keyword facilitates resource management by ensuring that the file is closed upon exiting the with statement. The with open(import_file, "r") as file: the open() function contains two parameters. The first involves identifying the file for import, while the second specifies the intended action with the file. In this context, "r" signifies my intention to read it. The code employs the as keyword to assign a variable named file, which stores the output of the .open() function while I work within the with statement.
Read the file contents
With the .read() method, I converted the file into a string so I could read what was inside it.
I can call the .read() function in the body of the with statement when I use an .open() function with the "r" argument for "read." I can read the file because the .read() method converts it into a string. I used the .read() method on the variable named file in the with statement. Then, I put the string that this method gave me into the variable ip_addresses.
To sum up, this code reads the "allow_list.txt" file and stores it as a string. I can then use that string to organize and get data from my Python program.
Convert the string into a list
I needed the allow list to be in list format so that I could remove certain IP addresses from it. In order to turn the ip_addresses string into a list, I next used the .split() method:
Appending the .split() function to the end of a string variable calls it. It turns the things that are in a string into a list. It's easier to remove IP addresses from the allow list when ip_addresses is broken up into a list. When called by itself, the .split() function splits text by whitespace into list elements. In this algorithm, the .split() function takes the data in the variable ip_addresses, which is a string of IP addresses with spaces between them, and converts it into a list of IP addresses. I moved this list back to the variable ip_addresses so that it could be stored.
Iterate through the remove list
One important part of my algorithm is iterating through the IP addresses that are in the remove_list. In order to do this, I used a for loop:
Python's for loop repeats code for a specified sequence. The for loop in this Python algorithm serves the purpose of applying specified code statements to all elements in a sequence. The for keyword begins the for loop. It is followed by the loop variable element and the keyword in. The keyword in means to iterate through the sequence ip_addresses and assign each value to the loop variable element .
Remove IP addresses that are on the remove list
The procedure necessitates the elimination of any IP address from the allow list, ip_addresses, that is also included in remove_list. Due to the absence of duplicates in ip_addresses, I was able to use the following code to do this:
First, I created a conditional to my for loop that checked to see if the loop variable element was in the ip_addresses list. It would have been wrong to use .remove() on elements that weren't in ip_addresses, so I did this.
Then I used .remove() on ip_addresses within that conditional. It was because I used the loop variable element as an argument that every IP address in the remove_list would be removed from ip_addresses.
Update the file with the revised list of IP addresses
As the last step in my algorithm, I had to update the allow list file with the revised list of IP addresses. Before I could do that, I had to convert the list back into a string. This is what I did with the .join() method:
All of the items in an iterable are put together into a string by the .join() method. I used the .join() method on a string that has characters that will break the items in the iterable once they are joined together. To write to the file "allow_list.txt", I used the .write() method and the .join() method to turn the list of ip_addresses into a string that I could pass to the .write() method as an argument. I used the string " " as a space to tell Python to use it to separate every element.
Another with statement and the .write() method were then used to update the file:
I used a second argument of "w" to the open() function in my with statement this time. I want to open a file and write over its contents, which is what this argument indicates. I can call the .write() function in the body of the with statement when this argument "w" is used. The .write() function replaces any existing file content with string data that is written to a specified file.
I wanted to write the updated allow list as a string to the file "allow_list.txt" in this case. This means that IP addresses that have been removed off the allow list will no longer be able access the restricted content. I appended the .write() function to the file object file that I identified in the with statement to rewrite the file. In order to specify that the contents of the file specified in the with statement should be replaced with the data in the ip_addresses variable, I passed it in as the argument.
Summary
An algorithm is created to eliminate IP addresses specified in a remove_list variable from the "allow_list.txt" file containing approved IP addresses. The algorithm consisted of opening the file, converting it to a string for reading, and subsequently converting this string into a list stored to the variable ip_addresses. I subsequently iterated through the IP addresses in the remove_list. In each iteration, I assessed whether the element belonged to the ip_addresses list. I applied the .remove() method to eliminate the element from ip_addresses. Subsequently, the .join() method was used to convert theip_addresses into a string, enabling the overwriting of the "allow_list.txt" file with the updated list of IP addresses.